Integrate Checkout on your website
This guide is for developers who want to add online payments to their website using Checkout.
Before you start
Before you start, you need:
- An Checkout Portal account. See create account for more information.
- Your integration keys for the website you are developing. Need help?
- A running web server which can host HTML pages and execute server side scripts.
What you are building
This guide shows you how to integrate an embedded checkout on your website. The image below shows the relationships between the customer, your website, and Checkout.
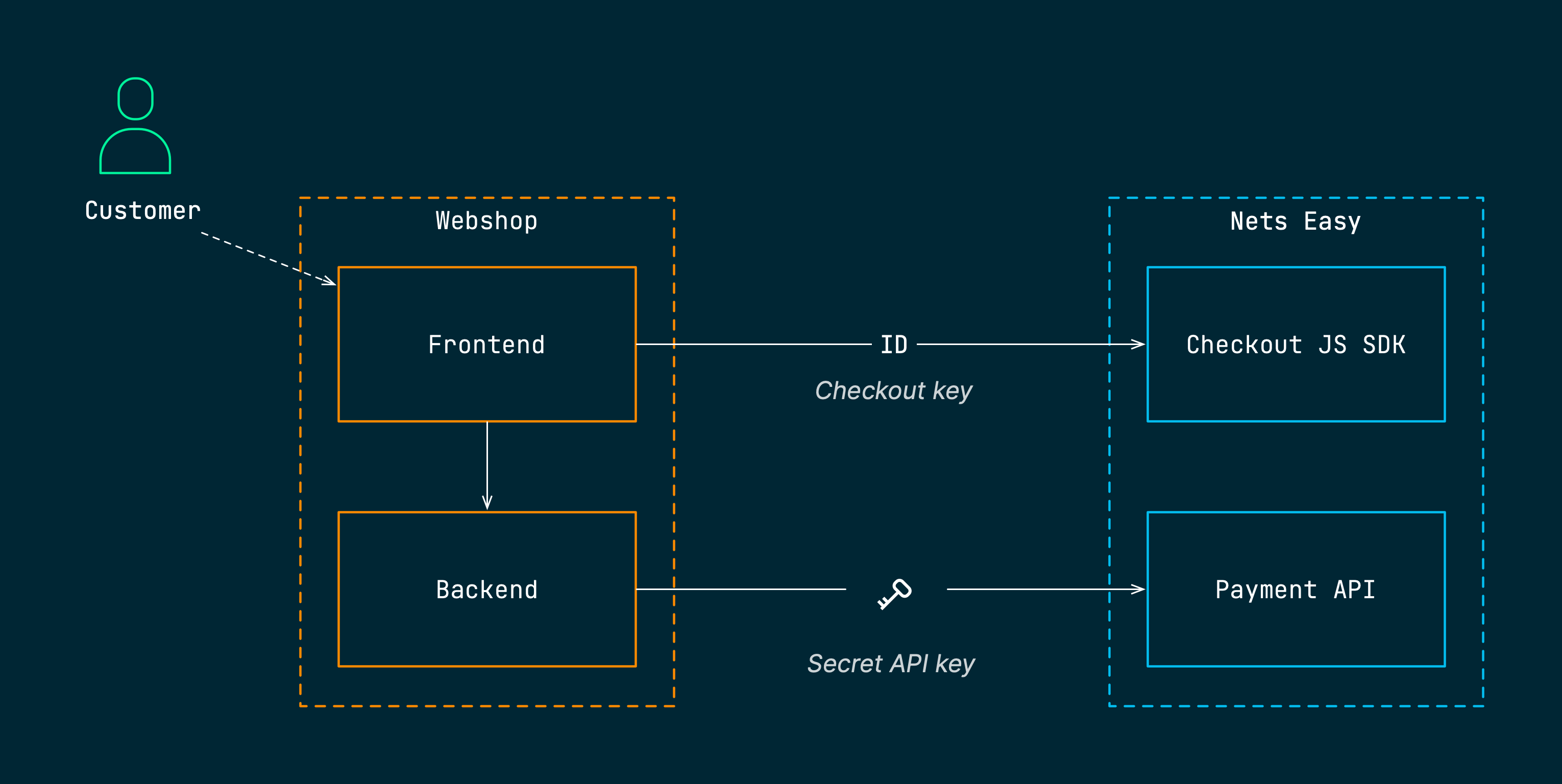
The customer interacts with your website exclusively when using an embedded checkout. The backend of your website communicates with Nexi Group over the RESTful Payment API using your secret API key. The frontend of your website uses the Checkout JS SDK, provided by Nexi Group, to handle the API integration with Checkout. The frontend of your site uses the checkout key to identify your webshop when communicating with Nexi Group.
Important
The secret API key must never be exposed to the public and should only be passed over encrypted server-to-server communication (HTTPS). In contrast, the checkout key is only an identifer and can be exposed to the frontend of your site.
Step 1: Initiate the checkout from your site (frontend)
The checkout is initiated from the client. To start implementing the checkout flow from the frontend, you need to add:
- A button that will allow the customer to initiate the checkout
- A JavaScript event handler attached to the button
The minimal shopping cart below will serve as a starting point for the payment flow:
Shopping cart
- PHP
- Node
<!DOCTYPE html> <html> <head> <title>Shopping Cart</title>
When you click the checkout button, the event handler sends an asynchronous request over HTTP(S) to the backend of your site. If you try clicking the checkout button now, you will receive an error since you haven't implemented the backend yet. Let's move on to the next step and fix this error.
Step 2: Create a payment object (backend)
Each payment session is represented by a payment object. In order to start a checkout flow for your customer, you first need to create a payment object and retrieve the paymentId
referencing that object. Creating a payment object requires your secret API key. Therefore, this request has to be initiated from the backend of your site.
The following backend script will create a new payment object and return the paymentId
to the frontend:
Create payment
- PHP
- Node
<?php $payload = file_get_contents('payload.json'); assert(json_decode($payload) && json_last_error() == JSON_ERROR_NONE);
Replace the text <YOUR_SECRET_API_KEY>
with your secret API key.
In this example we simply read a hardcoded JSON object from the file payload.json
shown below.
Normally, this would be the place where you dynamically create a JSON object based on your customer's order items.
For now, you can just add the static JSON file payload.json
that can be used for testing:
Request body
{ "checkout": { "integrationType": "EmbeddedCheckout", "url": "https://<YOUR_SERVER>/checkout.html",
The request body includes:
- Integration type: Either an embedded checkout that uses an
iframe
on your page or a pre-built checkout page hosted by Nexi Group that the customer will be redirected to. - Checkout URL: The URL to your checkout page. This URL must match the URL to your checkout page exactly, including protocol (http/https) and the fully qualified domain name (FQDN).
- Terms URL: A URL to a page on your site that describes the payment terms for your webshop.
- Order details: Includes order items, total amount, and currency
Make sure you replace the line:
"url": "https://<YOUR_SERVER>/checkout.html"
with the exact URL to the checkout page (created in the next step), or the page will fail to load later on. Verify the protocol (http/https), server address, and port you are using.
This is a basic example just to get you started. There are numerous settings you can make when creating or updating a payment object that are not covered here. See the guide Add shipping cost and the Payment API reference for more information.
You should now be able to click the checkout button and then see the paymentId
printed to the JavaScript console in your web browser.
Troubleshooting
If the paymentId
is not printed to the JavaScript console, you can also try loading the URL to the backend script (create payment) directly in your web browser.
That way, you can read the error messages outputted from the script more easily.
After the backend is implemented, it's time to go back to the frontend code and use the paymentId
to create the checkout page with the payment view.
Step 3: Add a checkout page (frontend)
It's time to create the HTML page that will embed the checkout iframe
. Add the following HTML code into a new file:
Checkout
<!DOCTYPE html> <html> <head><title>Checkout</title></head> <body>
Note a few things about the HTML code:
- The container element
<div id="checkout-container-div">
is the place where the checkoutiframe
will eventually be embedded. - Two JavaScripts are loaded:
checkout.js
from Nexi Group and the customscript.js
which you will implement in the next step. - Since this guide uses the Easy test environment,
checkout.js
is loaded fromtest.checkout.dibspayment.eu
. When using the live environment, you should instead usecheckout.dibspayment.eu
.
The checkout.html
page should always be requested with a URL parameter called paymentId
. This is because the paymentId
is needed in order to identify the current payment session when communicating with Nexi Group.
If the paymentId
is solely stored in the session, it might be lost during redirect to and from the checkout URL for certain payment methods when using our embedded checkout.
To prevent this, we include the query parameter when returning from a third party back to the checkout URL. This requires the checkout to look for the query parameter paymentId
and be able to initialize the checkout using the value specified.
Step 4: Generate checkout iframe using the Checkout JS SDK
It's time to create the JavaScript that will communicate with Checkout using the Checkout JS SDK.
Create a new file and add the following JavaScript code:
Checkout
document.addEventListener('DOMContentLoaded', function () { const urlParams = new URLSearchParams(window.location.search); const paymentId = urlParams.get('paymentId'); if (paymentId) {
Replace <YOUR_CHECKOUT_KEY>
with your checkout key for the test environment.
For the checkout.js
script to load correctly, it's important that you have specified the correct URL to your checkout page in Step 3.
The checkout object will trigger the event 'payment-completed'
once the payment has been completed. The callback function we provide navigates to completed.html
which you will create in the next step.
Troubleshooting
- Make sure you are using the correct integration keys for the test environment.
- For the
checkout.js
script to load correctly, make sure you have specified the correct URL to your checkout page. See Step 3.
Step 5: Add a "Order success" page
Add the following code to your site:
Order success
<!DOCTYPE html> <html> <head> <title>Payment completed</title>
That's it! It's time to test and verify that your new embedded checkout page is working.
Step 6: Test your checkout page
You should now have a rudimentary checkout page that can be tested using the test cards available on the page Test card processing.
Here's how you test your new checkout page:
- Reload the shopping cart page and click the "Proceed to Checkout" button.
- Fill out the address form using your email, phone, and postal code.
- Insert any of the sample card numbers and click the "Pay" button.
- Play around!
The simulated payment processing should now start and eventually get you back to the "Payment completed" page you created in the previous step.
Troubleshooting
- Verify that you are using the correct integration keys for the test environment.
checkout.js
will fail to load unless you provide the correct checkout URL in the JSON body specified in Step 2 when creating thepaymentId
.- When changing the checkout URL, make sure you reload the shopping cart page so that a new payment session is created (and a new
paymentId
).
Congratulations!
You've now integrated an embedded checkout.